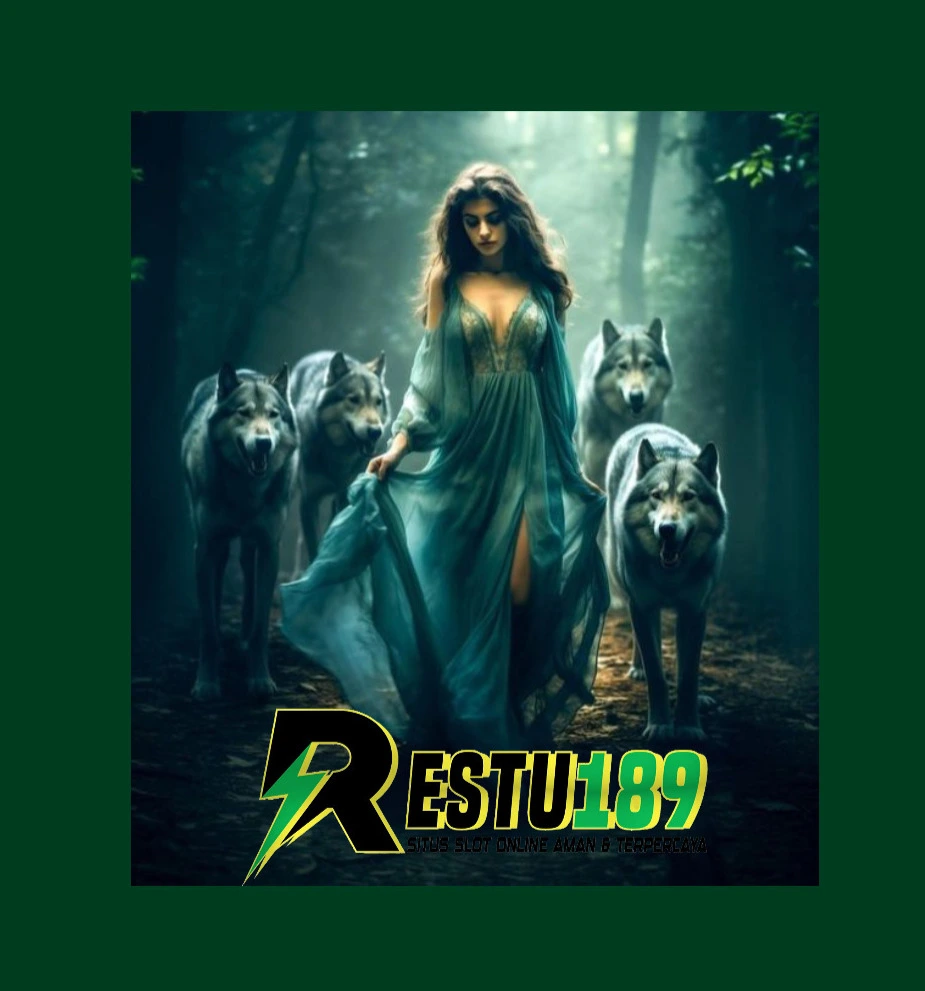
๐๐๐๐๐๐๐๐ || ๐๐๐๐ญ๐๐ซ ๐๐ค๐ฎ๐ง ๐๐๐ซ๐ฆ๐๐ข๐ง ๐๐๐ซ๐ฅ๐๐ฒ ๐๐๐ซ๐ฎ
๐๐ค๐ฌ๐๐ฌ ๐ฆ๐ฎ๐๐๐ก ๐๐๐ง ๐๐ฆ๐๐ง ๐ค๐ ๐ญ๐๐ซ๐ฎ๐ก๐๐ง ๐ฉ๐๐ซ๐ฅ๐๐ฒ ๐๐จ๐ฅ๐ ๐๐๐ง ๐๐๐ซ๐๐๐ ๐๐ข ๐ฃ๐๐ง๐ข๐ฌ ๐ญ๐๐ซ๐ฎ๐ก๐๐ง ๐จ๐ฅ๐๐ก๐ซ๐๐ ๐, ๐ฆ๐๐ง๐ฃ๐๐๐ข ๐ฉ๐ข๐ฅ๐ข๐ก๐๐ง ๐ฎ๐ญ๐๐ฆ๐ ๐๐๐ ๐ข ๐ฉ๐๐ซ๐ ๐ฉ๐๐ฆ๐๐ข๐ง ๐ฒ๐๐ง๐ ๐ข๐ง๐ ๐ข๐ง ๐ฆ๐๐ซ๐๐ฌ๐๐ค๐๐ง ๐ฌ๐๐ง๐ฌ๐๐ฌ๐ข ๐ญ๐๐ซ๐ฎ๐ก๐๐ง ๐ฉ๐๐ซ๐ฅ๐๐ฒ ๐ฒ๐๐ง๐ ๐ฌ๐๐ซ๐ฎ ๐๐๐ง ๐ฆ๐๐ง๐ ๐ฎ๐ง๐ญ๐ฎ๐ง๐ ๐ค๐๐ง.